# 代码简洁和代码优雅
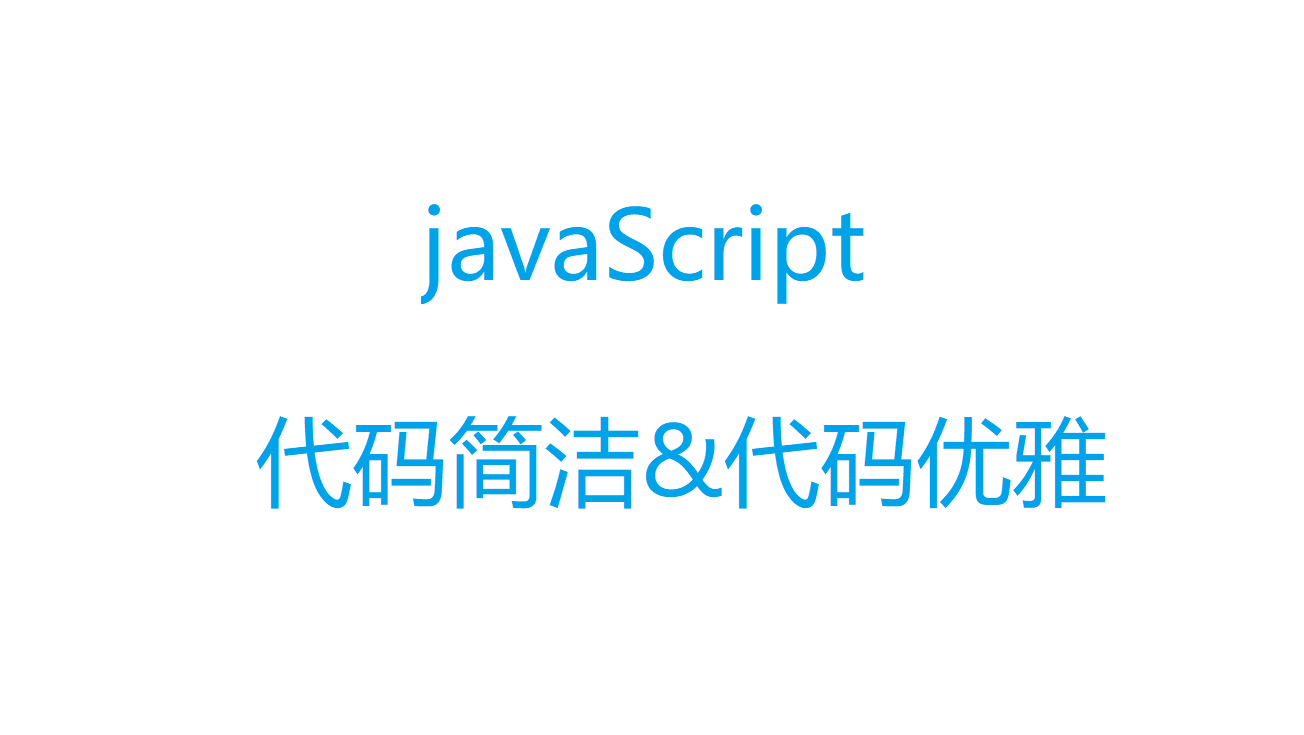
# 接口返回数据判断
- 错误前置,对接口返回数据做错误前置阻断,特别是在场景复杂的时候优化效果更明显
const { code = 1, data = {} } = api();
// bad
if (code === 0) {
this.data = data;
}
//good
if (code !== 0 || data == null) return;
this.data = data;
- async/await 更优雅,合理使用可选链操作符和空值合并运算符,同时合理解构,并且做好数据兜底
//bad
this.list = api().then((res) => {
if (res.data && res.data.second) {
this.list = res.data.second.list;
}
});
//good
const { code = 1, data = {} } = await api();
if (code !== 0) return;
const { list } = data.second ?? [];
this.list = list;
# 简单的 if-else 判断使用三目运算,布尔类型的判断可直接拿判断条件来赋值(不确定的情况下可以!!取反)
//bad
if (value === 0) {
this.label = '成功';
} else {
this.label = '失败';
}
//good
this.label = value === 0 ? '成功' : '失败';
//bad
if (ifShow === 1) {
this.show = true;
} else {
this.show = false;
}
//good
this.show = ifShow === 1;
//bad
if (arr.length === 0) {
this.show = true;
} else {
this.show = false;
}
//good
this.show = !!arr.length;
# 正确使用数组的方法并结合&&
//bad
if (a === 1 || a === 2 || a === 3 || a === 4) {
this.result = a;
}
//good
[1, 2, 3, 4].includes(a) && this.result = a;
const numbers = [1, 2, 3, 4];
//bad
for (let item of numbers) {
this.result += item;
}
//good
this.result = numbers.reduce((total, item) => total + item, 0);
//bad
const numbers = [1, 2, 3, 4];
for (let index in numbers) {
this.entries[index] = numbers;
}
//good
this.entries = numbers.map((x) => x);
const list = [1, 2, 3, 4, 'a'];
let hasString = false;
let numberList = [];
let allNumber = true;
//bad
for (let item of list) {
if (item === +item) {
numberList.push(item);
} else {
allNumber = false;
}
if (typeof x == 'string') {
hasString = true;
}
}
//good
hasString = list.some((x) => typeof x == 'string');
numberList = list.filter((x) => item === +item);
allNumber = list.every((x) => item === +item);
# 使用策略模式优化代码
//bad
<template>
<el-button disabled="cardStatus === 0 || cardStatus === 1">查看分析核实</el-button>
<el-button disabled="cardStatus === 2">修改分析核实</el-button>
<el-button disabled="eocStatus === 3">推送EOC</el-button>
</template>
//good
<template>
<el-button v-for="item in btnList" key="item.label" disabled="item.disabled">{{item.label}}</el-button>
</template>
<script>
export default {
data(){
retrun {
btnList:[
{label:'查看',disabled:[0,1].includes(cardStatus)},
{label:'修改',disabled:cardStatus===2},
{label:'推送',disabled:cardStatus===3},
]
}
}
}
</script>
let statusText = '暂无状态';
//bad
if (status === 0) {
statusText = '已取消';
} else if (status === 1) {
statusText = '已提交';
} else if (status === 2) {
statusText = '已变更';
}
//good
const textList = ['已取消', '已提交', '已变更'];
statusText = textList[status] ?? statusText;
//bad
if (code === 'get') {
this.getList();
} else if (code === 'set') {
this.setVal();
} else if (code === 'cancel') {
this.cancelFn();
}
//good
const valToFn = {
get: this.getList,
set: this.setVal,
cancel: this.cancelFn,
};
valToFn[code]();
# 尽量使用纯函数,参数过多时应设置为对象,同时需要做好参数校验或者给参数默认值
相同的入参,返回的结果始终一致,同时尽量避免修改入参
const list = ['a', 'b', 'c', 1];
//bad
function fn(list, filter, params) {
!filter &&
list.push({
params,
data: new Date(),
});
return list;
}
//good
function addParams({ list, filter = false, params }) {
if (!params) throw new Error('参数不能为空');
return filter
? list
: list.concat({
params,
});
}
# 对逻辑复杂的代码做好注释,对一些枚举值、常量做好归类注释
- 风骚的代码也许让我们当时成就感满满,但是不做好注释,后期回过头来还是需要花时间去梳理,好记性不如烂笔头,与其相信自己能在几个月后还记得代码逻辑,还不如把自己当时的想法或者业务逻辑注释上去
- 对于一些函数或者变量命尽可能做到顾名思义
# 感谢各位看官老爷!
附:收藏一些不错的文章